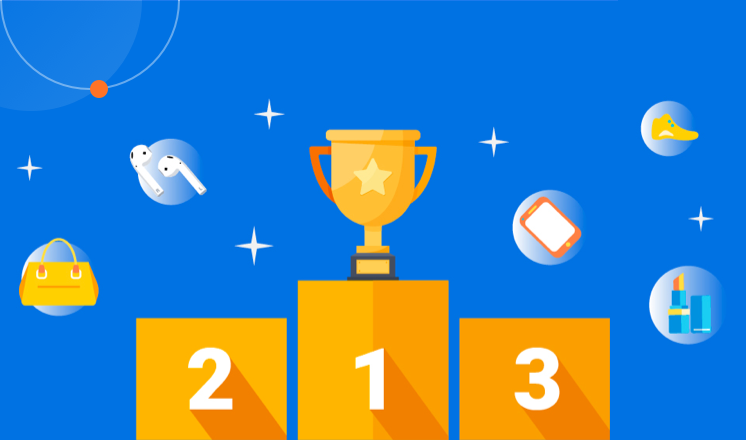
When it comes to web scraping and data collection, using a proxy rotator is crucial to avoid IP bans and access restrictions. In this article, we will explore the use of the requests-ip-rotator library to build a robust proxy rotator in Python. We will cover the basics of setting up a proxy server in Python, integrating the proxy rotator, and utilizing it to make requests through a pool of rotating proxies.
The requests-ip-rotator is a powerful tool that allows us to easily manage and rotate proxies within our Python applications. By leveraging this library, we can create a seamless experience for web scraping tasks, ensuring that our requests are routed through diverse IP addresses to avoid detection and maintain anonymity.
To get started, we first need to install the requests-ip-rotator library using pip:
```python
pip install requests-ip-rotator
```
Once the library is installed, we can begin implementing the proxy rotator in our Python code. We'll initialize the rotator, add a list of proxy IP addresses and ports, and configure the rotation settings. With just a few lines of code, we can set up a reliable proxy rotator that automatically switches between proxies for each request.
```python
from requests_ip_rotator import ProxyList
proxy_list = [
{'ip': '123.456.789.001', 'port': 8080},
{'ip': '123.456.789.002', 'port': 8080},
# Add more proxies as needed
]
rotator = ProxyList(proxy_list)
rotator.set_rotation_settings({'rotating': True, 'change_after': 3})
```
With the proxy rotator configured, we can now use it with the requests library to make HTTP requests through rotating proxies. This allows us to scrape data from websites without the risk of being blocked or identified by our IP address.
```python
import requests
url = 'https://example.com'
response = rotator.get(url)
print(response.text)
```
In addition to using a predefined list of proxies, the requests-ip-rotator also supports fetching proxies from free proxy rotator services. By integrating these services, we can further enhance the diversity and reliability of our proxy pool.
Overall, the requests-ip-rotator library provides a convenient and effective solution for managing proxy rotation in Python. Whether it's for web scraping, data collection, or anonymity preservation, incorporating a proxy rotator into your Python projects can significantly improve their performance and reliability.