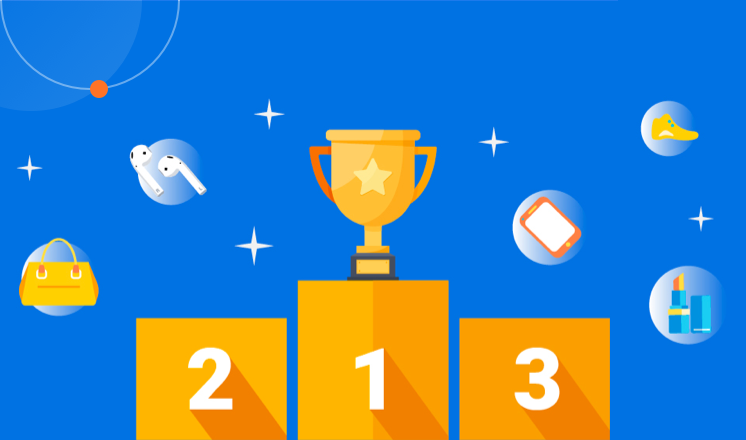
When it comes to web scraping with Python, using a rotating proxy with Selenium can be a game changer. In this article, we will explore the concept of rotating proxies and how to integrate them with Selenium for efficient and anonymous web scraping.
What is a Rotating Proxy?
A rotating proxy is a type of proxy server that automatically changes the IP address it uses for each web request. This rotation of IP addresses helps in avoiding IP bans and rate limits imposed by websites during web scraping. By constantly changing the IP address, rotating proxies allow you to scrape large amounts of data without getting blocked.
Using Rotating Proxy with Selenium in Python
To use rotating proxies with Selenium in Python, you can make use of libraries such as `selenium` and `requests`. Additionally, you can utilize proxy services that offer rotating IP addresses. Here's a basic example of how you can integrate rotating proxies with Selenium in Python:
```python
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
# Set up rotating proxy
proxy = 'your_rotating_proxy_address'
# Set up Chrome options
chrome_options = Options()
chrome_options.add_argument('--proxy-server=%s' % proxy)
# Initialize Chrome driver with the configured proxy
driver = webdriver.Chrome(options=chrome_options)
# Now you can use the driver for web scraping with rotating proxy
```
By setting up the Chrome driver with the configured rotating proxy, you can now scrape websites using Selenium while the IP address is automatically rotated for each request. This can be extremely useful for scraping data from websites that have strict anti-scraping measures in place.
Benefits of Using Rotating Proxy with Selenium
There are several benefits of using rotating proxies with Selenium for web scraping in Python. Some of the key advantages include:
1. Anonymity: Rotating proxies help in maintaining anonymity while scraping websites, as the IP address keeps changing.
2. Avoiding Blocks: With rotating proxies, you can avoid getting blocked by websites that impose IP bans or rate limits.
3. Scalability: Rotating proxies allow for scalable web scraping by enabling the scraping of large amounts of data without interruptions.
Conclusion
In conclusion, using rotating proxies with Selenium in Python can greatly enhance the efficiency and effectiveness of web scraping. By leveraging rotating proxies, you can scrape websites anonymously and at scale, while avoiding common issues such as IP bans and rate limits. If you're looking to level up your web scraping game, integrating rotating proxies with Selenium is definitely worth considering.