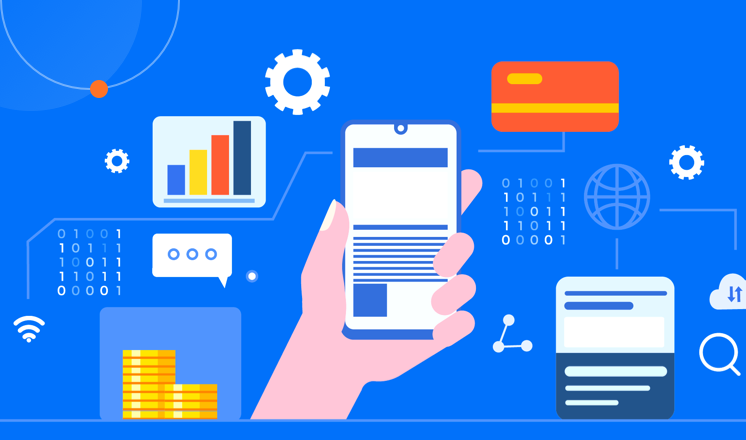
Scraping Twitter data using Python is a common task for many data analysts and researchers. However, due to Twitter's strict rate limits and anti-scraping measures, it can be challenging to efficiently gather large amounts of data without getting blocked. One effective way to overcome these challenges is by using proxies in your web scraping process. In this article, we will discuss how to scrape Twitter data using Python with the help of proxies, and how it can improve the efficiency and reliability of your data gathering process.
## Understanding the Need for Proxies
Before we dive into the specifics of using proxies for scraping Twitter data, let's first understand why they are necessary. Twitter, like many other websites, employs rate limits and anti-scraping techniques to prevent automated bots from accessing and gathering data from its platform. When you make a large number of requests to Twitter from a single IP address, you risk triggering these rate limits and getting your IP banned.
Proxies provide a solution to this problem by allowing you to make requests from multiple IP addresses, effectively distributing the traffic and reducing the likelihood of triggering rate limits or getting blocked. By rotating through a pool of proxies, you can scrape Twitter data at a larger scale while minimizing the risk of being detected as a bot.
## Setting Up a Proxy for Scraping Twitter Data
There are various ways to use proxies for scraping Twitter data in Python. One popular approach is to use the `requests` library along with a proxy service to make HTTP requests through different IP addresses. Here's a simple example of how you can set up a proxy for your scraping script:
```python
import requests
proxy = {
'http': 'http://your-proxy-ip:port',
'https': 'https://your-proxy-ip:port'
}
response = requests.get('https://twitter.com', proxies=proxy)
print(response.text)
```
In this example, we define a proxy dictionary containing the IP address and port of the proxy server, and pass it to the `requests.get` method to make the HTTP request through the specified proxy. Keep in mind that you will need to use a reliable proxy service that provides high-quality, non-blacklisted proxies for this to work effectively.
## Best Practices for Using Proxies
While using proxies can be beneficial for scraping Twitter data, it's important to follow best practices to ensure the success of your scraping efforts. Here are some tips to keep in mind:
1. **Use Residential Proxies**: Residential proxies, which are IP addresses assigned to homeowners by Internet Service Providers, are often more reliable and less likely to be detected as proxies by websites like Twitter.
2. **Rotate Proxies**: To avoid being flagged for suspicious activity, regularly rotate through a pool of proxies to distribute your requests across different IP addresses.
3. **Monitor Proxy Performance**: Keep an eye on the performance of your proxies to identify any slow or non-responsive IP addresses, and replace them with better alternatives.
4. **Respect Twitter's Robots.txt**: Before scraping Twitter data, review Twitter's `robots.txt` file to understand any restrictions or guidelines for web crawlers and bots.
By following these best practices, you can maximize the effectiveness of using proxies for scraping Twitter data and minimize the risk of encountering blocks or bans.
## Conclusion
In conclusion, using proxies is a valuable technique for scraping Twitter data with Python. By leveraging proxies, you can enhance the scalability and reliability of your web scraping process while mitigating the risk of being blocked by Twitter's anti-scraping measures. Whether you are conducting social media analysis, sentiment analysis, or research, understanding how to use proxies for scraping Twitter data is a valuable skill that can elevate your data gathering capabilities.