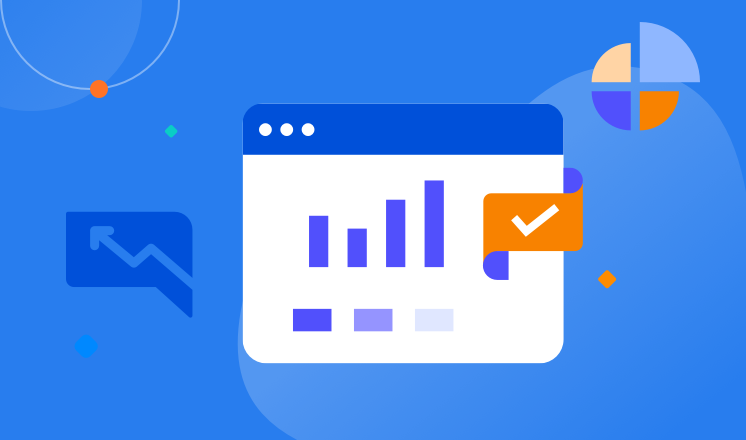
The Power of Proxy in Python: Leveraging Py Socks and Py Proxy
In the world of web scraping and data gathering, the use of proxies is essential for maintaining anonymity, bypassing restrictions, and accessing geo-blocked content. Python, being a versatile programming language, offers several libraries and tools for working with proxies, including Py Socks and Py Proxy.
Py Socks is a Python library that enables you to establish a connection through a SOCKS proxy, allowing you to route your traffic through a different IP address. This can be particularly useful when dealing with websites that impose restrictions based on IP addresses or when you need to access content that is not available in your region.
To leverage Py Socks in your Python projects, you can simply install the library using pip:
```bash
pip install pysocks
```
Once installed, you can use Py Socks to create a proxy connection in your Python code. For example, the following snippet demonstrates how to make a request using the requests library through a SOCKS proxy:
```python
import requests
import socks
import socket
socks.set_default_proxy(socks.SOCKS5, '127.0.0.1', 9050)
socket.socket = socks.socksocket
response = requests.get('https://example.com')
print(response.text)
```
In this example, we set the default proxy to use a SOCKS5 proxy located at '127.0.0.1:9050' and then make a GET request to 'https://example.com' using the requests library.
Similarly, Py Proxy is another Python library that provides a simple interface for working with HTTP and HTTPS proxies. It allows you to easily create proxy connections and integrate them into your web scraping and data gathering workflows.
To install Py Proxy, you can use pip:
```bash
pip install pyproxy
```
Once installed, you can use Py Proxy to create an HTTP or HTTPS proxy connection in your Python code. Here's an example of how you can utilize Py Proxy to make a request through an HTTP proxy:
```python
import pyproxy
proxy = pyproxy.Proxy('http', '127.0.0.1', 8888)
response = proxy.get('https://example.com')
print(response.text)
```
In this example, we create an HTTP proxy object that points to 'http://127.0.0.1:8888' and then make a GET request to 'https://example.com'.
By leveraging Py Socks and Py Proxy in your Python projects, you can enhance your web scraping and data gathering capabilities by effectively managing and rotating proxies, ensuring the reliability and efficiency of your data collection processes.
In conclusion, the use of proxies in Python, facilitated by libraries such as Py Socks and Py Proxy, opens up a world of possibilities for web scraping, data gathering, and online anonymity. Whether you need to access geo-blocked content, bypass IP restrictions, or simply protect your identity, Python provides the tools and resources to empower you in the realm of proxy usage.